.Net Core 2.2 Console Application - Autofac
C# .Net Core 2.2. Console Application using Autofac Dependency Injection with a ContainerBuilder Class
This article describes how to create a .Net Core 2.2 Console Application to generate a DataTable from a SQL Server data source and output the data to Standard Output (the Console Window). The console application uses a ContainerBuilder to register (expose) the Service and the Repository using their Interfaces in an Autofac ContainerBuilder class. The Main section of the Console app will set the DI Lifetime scope of the Service and resolve it for use in the project.
Create a C# .Net Core version 2.2 Console Application
Create a new C# Console Application in Visual Studio 2017 that will handle any errors thrown back by SQL Server (or in the code itself).
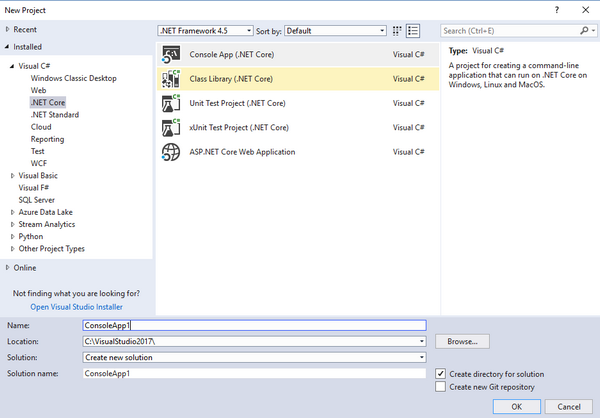
This application will require Interfaces and Classes for a Service and a Repository and a Class for the ConfigContainer. In the upper part of the code, add references to use the System.Data.SqlClient and the Configuration assemblies below.
Install NuGet Packages for Project
Add the following NuGet Packages from Tools, NuGet Package Manager, Manage NuGet Packages for Solution:
- Microsoft.Extensions.Configuration
- Microsoft.Extensions.Configuration.Abstractions
- Microsoft.Extensions.Configuration.Binder
- Microsoft.Extensions.Configuration.EnvironmentVariables
- Microsoft.Extensions.Configuration.FileExtensions
- Autofac
In the Solution menu, the NuGet Dependencies will appear as shown below.
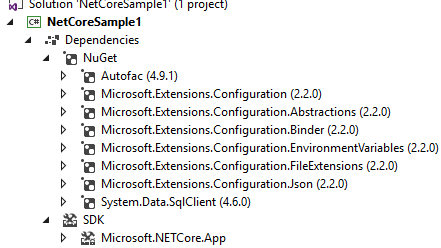
Create the DI Interfaces
Service Interface - Create an Interface for the service to Write the data and return a Dataset.
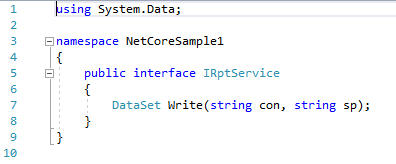
Repository Interface - Create an Interface for the repository to WriteData the data and return a Dataset.
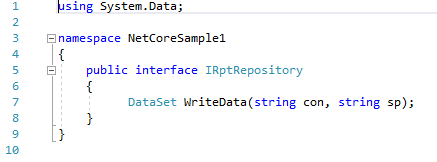
Create the DI Classes
Repository Class - Create a new Class that implements the Repository Interface with a generic Constructor and 2 variables for the SQL connection and the Stored Procedure name. Implement the Repository Interface member WriteData which will return a DataSet. Add an additional function to Get the Data for the DataSet.
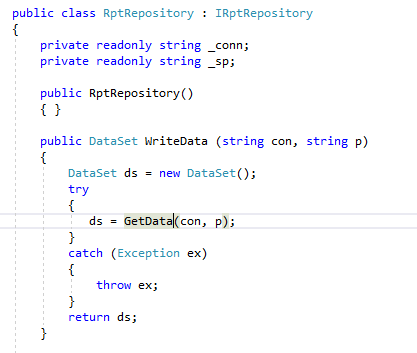
Additional DataSet Function - Add a Function GetData which takes the Sql Connection String and Stored Proc name to execute the SqlCommand and Fill the DataAdapter with the DataSet for the Repository.
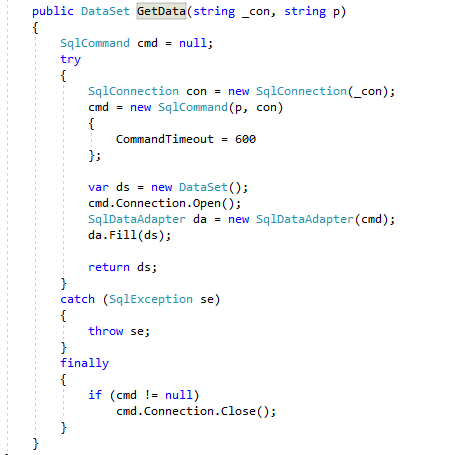
Service Class - Create a new Class that implements the Service Interface. Add a field of the local variable of the Interface Repository type. Create the required Service Interface member Write which calls the Repository Interface member WriteData to return the DataSet to Main in Program.cs.
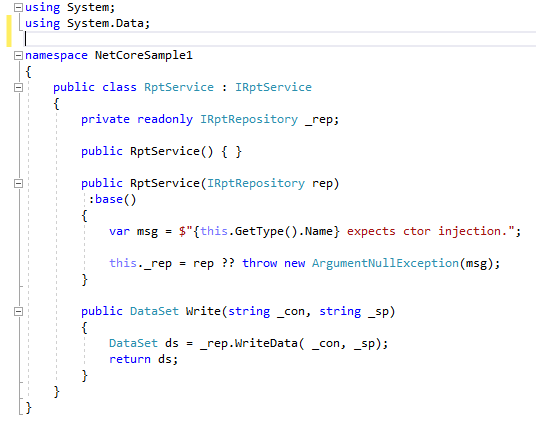
Create the Config Container
Create a Class named ContainerConfig and add code to the Static Configure Class to use a ContainerBuilder to Register both Repository and Service Classes and builds the Container with the registered components.
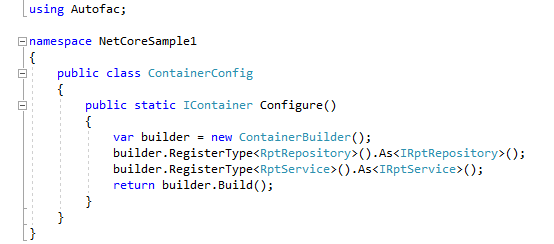
Creating the Main Code
ConfigurationBuilder Class - Add a JSON Configuration file to the project (appsettings.json). Add the ConnectionString and user Stored Proc name to the file.

In Main add the ConfigurationBuilder code and assign the config information from the Build() into a variable and extract each variable from the JSON file into local variables.
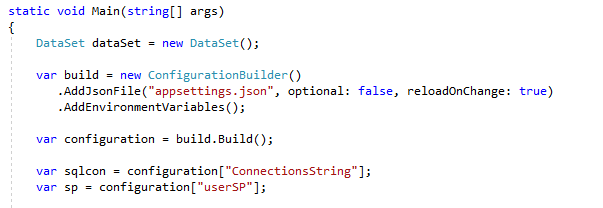
ContainerConfig Class Dependency Injection - Add code to Main to Configure the Container and extract the DataSet for processing by Main.
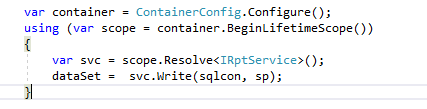
Data Output - The DataSet is converted to a DataTable and the Header Columns are extracted in the First foreach loop. The following foreach loop iterates through the Rows of the DataTable and concatenates the items in each column into a single row for output with a line break at the end.
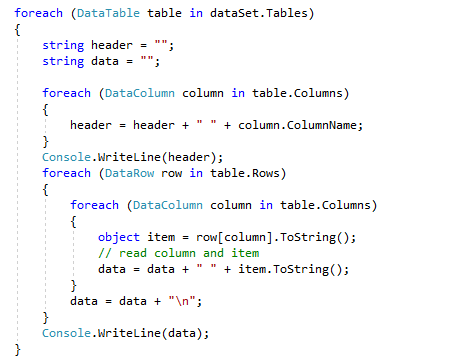
Build and Run the application to view the results.
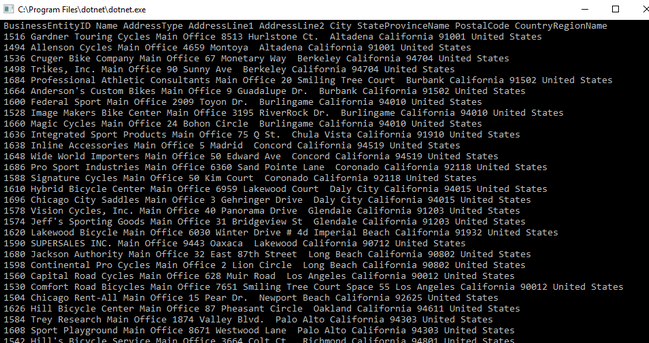