C Sharp Email Applications
Creating C# Email Applications in the Development Environment
This article describes how to develop an email application on a development PC without an SMTP server.
The current recommendation for Windows developers is to NOT create a local SMTP server (requiring punching a hole in the firewall and risking spam, etc.) when creating applications.
Rather than downloading, installing and configuring an email server (such as hMailServer), a domain, MX record settings, etc., there are alternatives to testing if the email is successfuly sent or not.
One way is to use a dummy SMTP tool (listening on Port 25) to detect if an email is successfully "sent". The tool used in this article is smtp4dev which can be found here: smtp4dev.com
Another way is to use an internet mail account with credentials to send the email to the user. I will be using my email account at msn (aka hotmail.com)
C# Program - Email using a Listener
Create a new Windows Project in Visual Studio .Net.
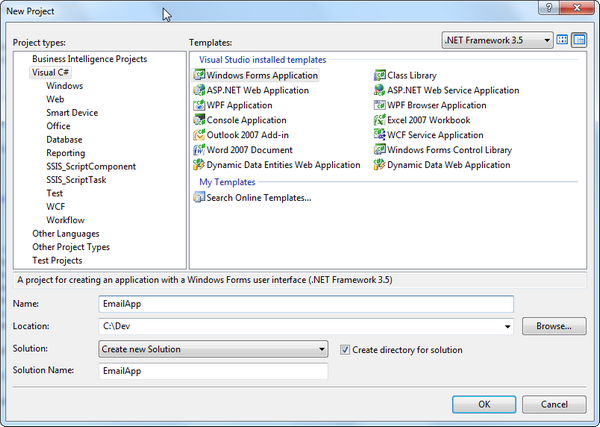
Create a new form and add Text Boxes and Labels and 2 Command Buttons.
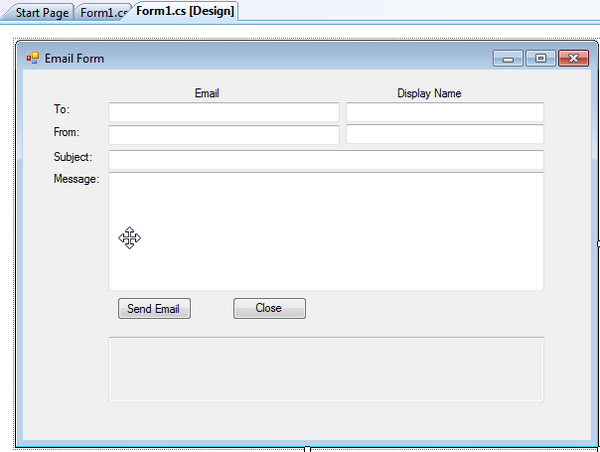
Add the following code to the top of the Form Code file:
using System.Net.Mail;
Add some code to the Send Email command button.
private void btnEmail_Click(object sender, EventArgs e)
{
try
{
MailMessage m = new MailMessage();
m.From = new MailAddress(txtFrom.Text, txtDisplayFrom.Text);
m.To.Add(new MailAddress(txtTo.Text, txtDisplayTo.Text));>
m.Subject = txtSubject.Text;
m.Body = txtMsg.Text;
SmtpClient client = new SmtpClient("localhost");
client.Send(m);
txtStatus.Text = "You have successfully sent the email. Thank you.";
}
catch (Exception ex)
{
txtStatus.Text = "Error: " + ex.Message;
}
}
Make sure smtp4dev is running and listening on Port 25 for email and run the application.
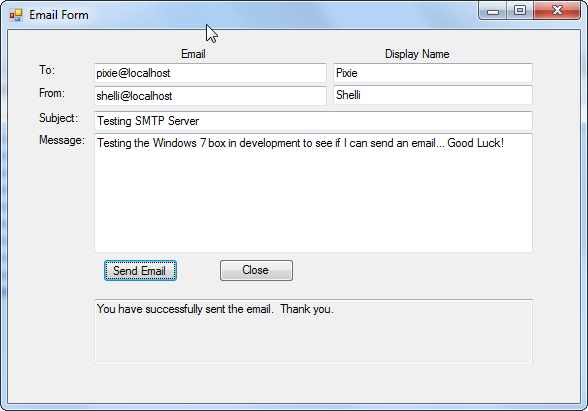
Check smtp4dev to see that the email was "sent" from the .Net Application.
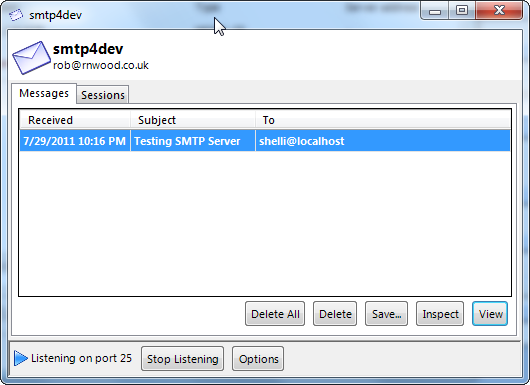
Highlight the message and click on Inspect to view the message.
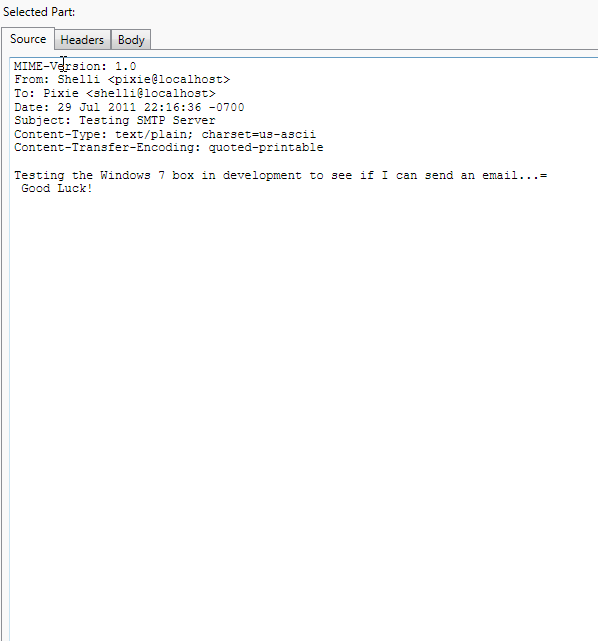
C# Program - Email using an Internet Account
To use an internet account, add this code to the top of the Code file:
using System.Net;
Change the code by commenting out the SmtpClient "localhost" line and adding the rest:
//SmtpClient client = new SmtpClient("localhost");
SmtpClient client = new SmtpClient("smtp.live.com", 587);
client.UseDefaultCredentials = false;
client.Credentials = new NetworkCredential("michelleaignacio@hotmail.com", "*****");
client.EnableSsl = true;
client.Timeout = 10000;
client.DeliveryMethod = SmtpDeliveryMethod.Network;
m.BodyEncoding = UTF8Encoding.UTF8;
m.DeliveryNotificationOptions = DeliveryNotificationOptions.OnFailure;
Run the application with the changes
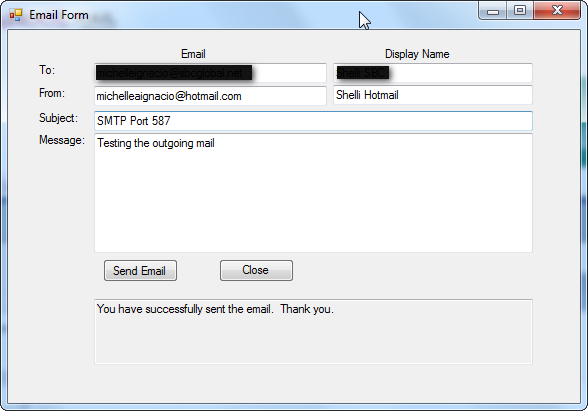
Check the internet accounts to see that the message was sent and received.
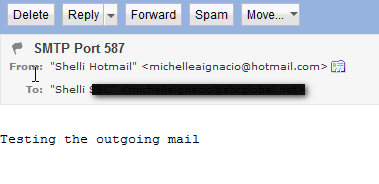
C# Program - Email and Special Characters
The subject line cannot contain any special characters or an error will be thrown.
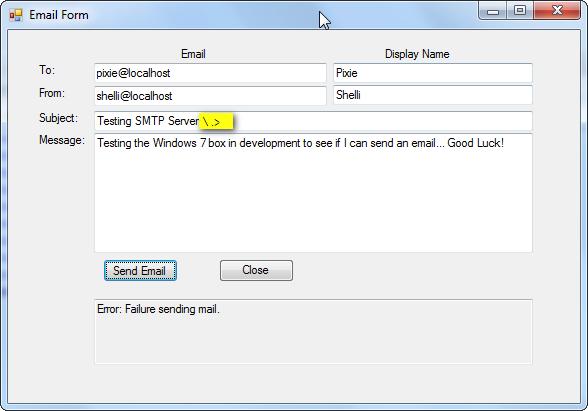
To get around this, at the top, add
using System.Text.RegularExpressions;
Change the line of code for the Subject to:
m.Subject = Regex.Replace(txtSubject.Text, "\\W", " ");
This will remove any "Special Characters" from the text.
C# Program - Email Attachments
To add attachments, the form can be modified to add an Attachment Path TextBox and a Button to Open a FileDialogBox to capture the path & location of the file to add to the email.
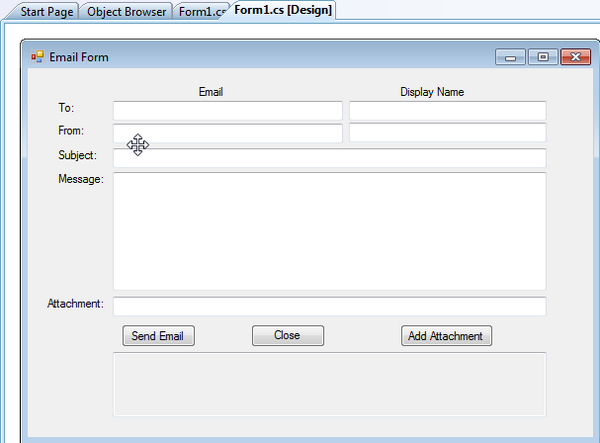
private void btnAttach_Click(object sender, EventArgs e)
{
OpenFileDialog fileDialog1 = new OpenFileDialog();
if (fileDialog1.ShowDialog() == DialogResult.OK)
{
if (fileDialog1.FileName != null)
{
txtAttach.Text = fileDialog1.FileName;
}
}
}
To add the attachment to the email, add the following code to the Send Email button code:
m.Attachments.Add(new Attachment(txtAttach.Text));
When the application is running, upon pressing the Add Attachment button, a dialog box will appear allowing the user to select the path to the file to upload.
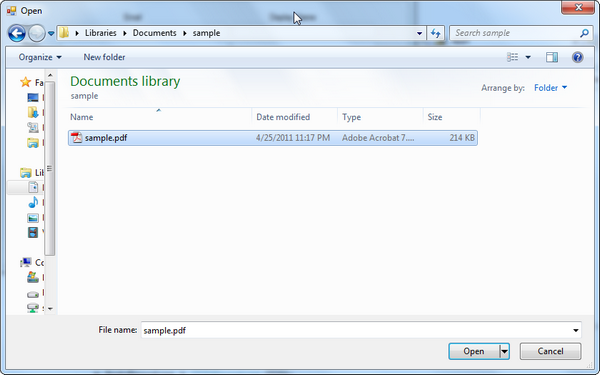
To send the email with the attachment, click on the Send Email button
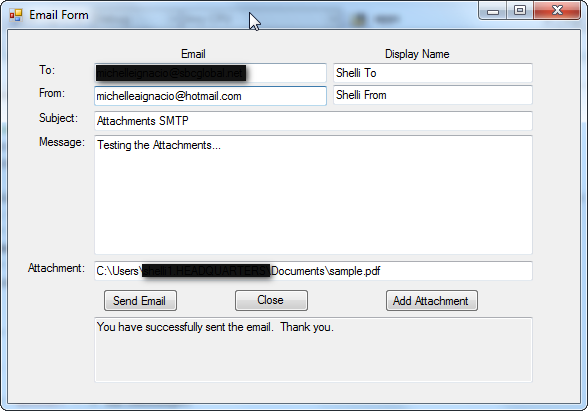
Verify that the email was sent with the attachment.
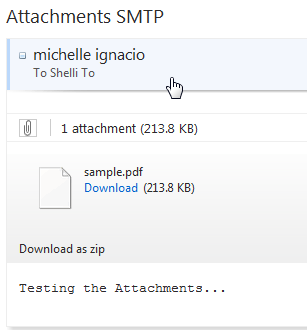